Java Basics 101
Lesson 3 - If Statements
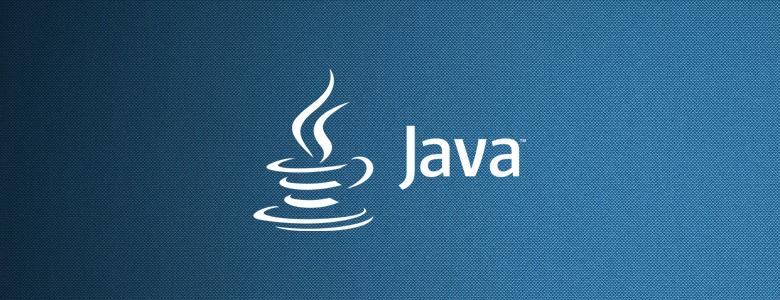
Now that we have covered the basics of variables, we can move onto if statements. So, what is an if statement? An if statement is a block of code that says, “If this condition is met, do something”. You are then to input a single or multiple conditions that need to be met.
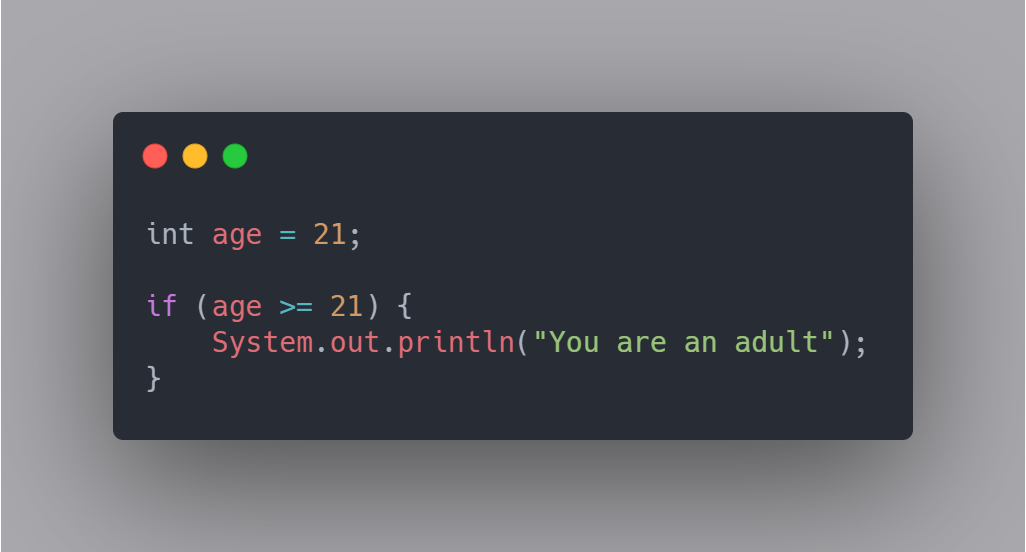
This can be viewed in a very basic sense or in a very complex sense depending on the conditions. A simple example would be the code above. The code above runs a conditional to see the the variable "age" is 18 or greater. If it is, like in this case, the
block surrounded by the brackets { }
will be executed. In this case it will just run a print statement telling us that we are indeed an adult. If we are not an adult, the program will
not print anything.
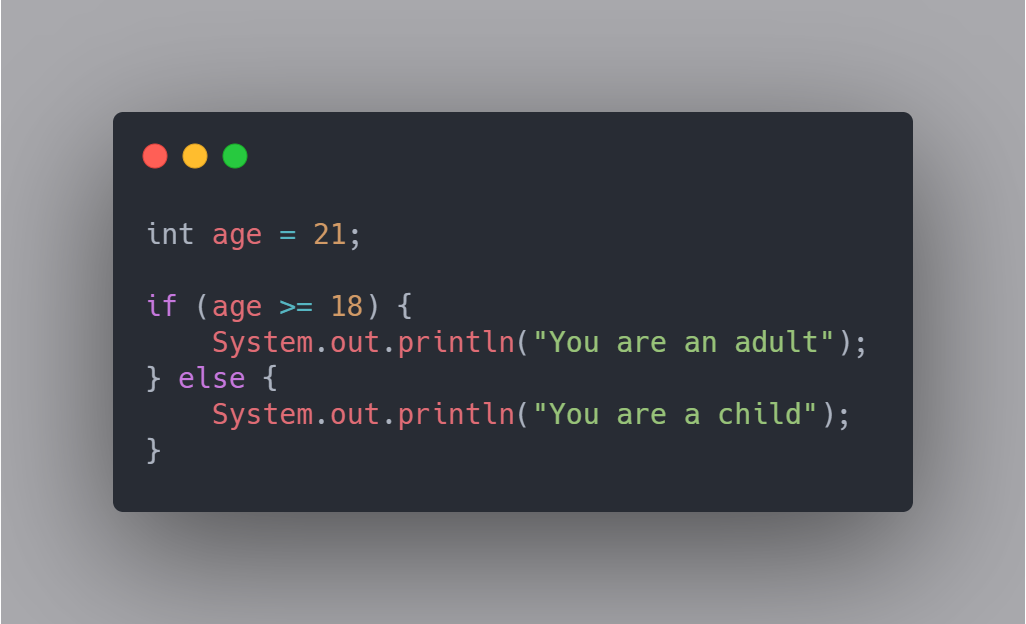
This is an example of an if/else statement. In the first example, we had a program that would print out that you are an adult if you are 18 or older. It didn't do anything if you were younger than 18 though. In this example, if you are not 18 or older,
it was automatically run the else
block and print out that you are a child.
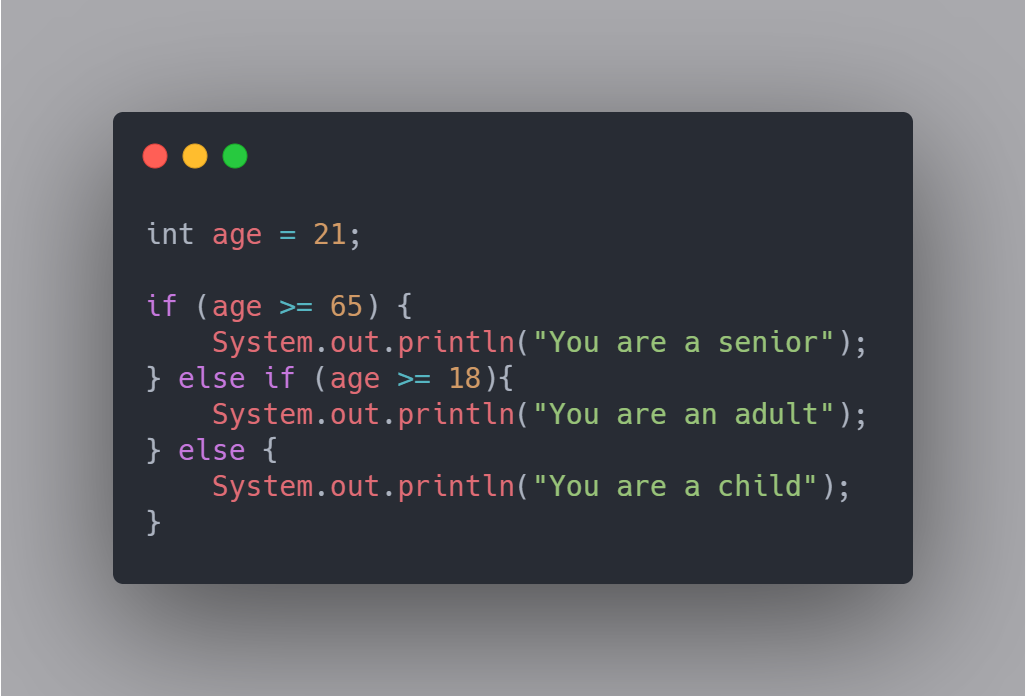
This is an example of an if/elseif/else statement. We have covered how blocks work in an if statement would if the returns true, it'll execute that block, otherwise it'll execute the else block. Now we see in this example, an else if
block. So what does an else if block do? If the first if statement returns false, it will look at the else if and see if that returns true or false now. If it returns true, it'll execute that block and exit the if statements.
If it returns false it will continue progressing through the else if blocks to see if any of them are true. If none are true, it'll execute the else statement if there is one.
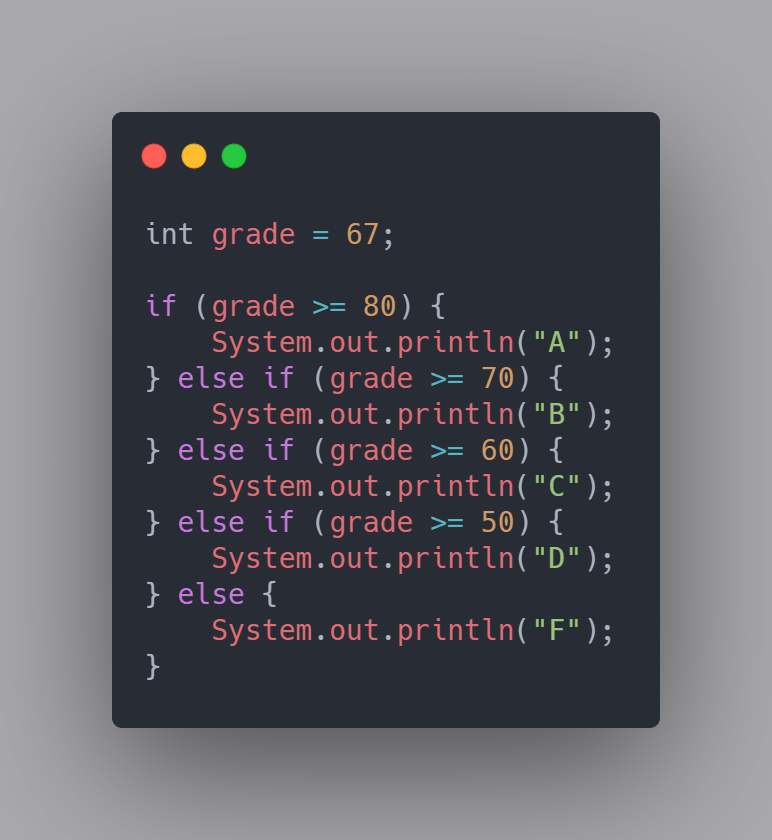
In this final example of if/elseif/else statements, we will look at a piece of code that will look at your percentage grade and return a letter grade. As you can see, we have the checks lining up in descending order so it will first check for the highest letter grade and work towards the lowest letter grade. This program will compare the student's grade to the 'A' category. the students grade is lower than 80, so the program moves into the first else if. The program then compares the students' grade to the 'B' category. The student's grade is lower than 70, so it moves onto the next else if. Then the program compares the student's grade to the 'C' category. Finally the student's grade is higher than the base of the 'C' category. The program prints out the 'C' grade and exits the if statements.
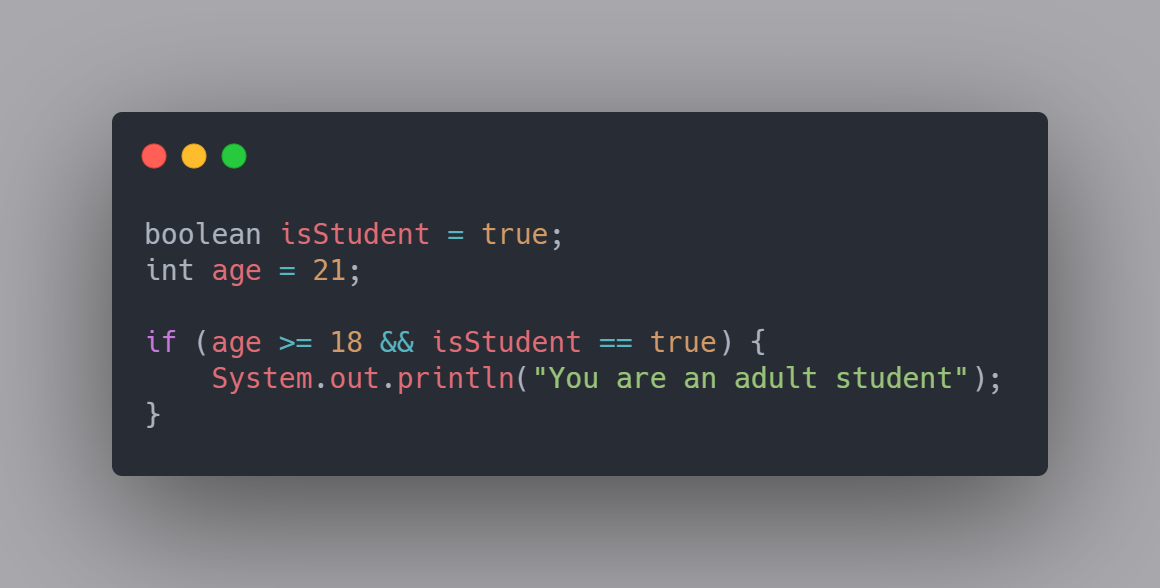
In this example, we are putting multiple seperate conditions inside of a single if statement. This code is saying that you must both be 18 or older, plus you must be a student for this print statement to run. There are two keys in multiple conditioned
if statements. There is the "And" operator, shown here as &&
. This means both statements must be true for the if block to execute. There is also the "Or" operator, shown here as ||
.
This means either statement has to be true for it to execute. The Or statement does not require both to be true.
The "Equals" operator ==
inside the if statement is not setting any value like the =
sign normally does. This double equals sign is checking to see if these values are equal. So in this case the program
is checking the isStudent
variable to see if it equals true. If the program was to check if they are not a student, it would use the "Not Equals" operator, which look like this !=
.
As a side note about the isStudent == true
check. This can be simplified to just isStudent
. This is because any boolean has to be either true or false. If you put this alone in an if statement, it
will check if the boolean is true and execute, or if it is false it can just skip. If we were looking for it to be false, we could put a "Not" operator !
infront to check. It would look like this inside the if
statement
!isStudent
.
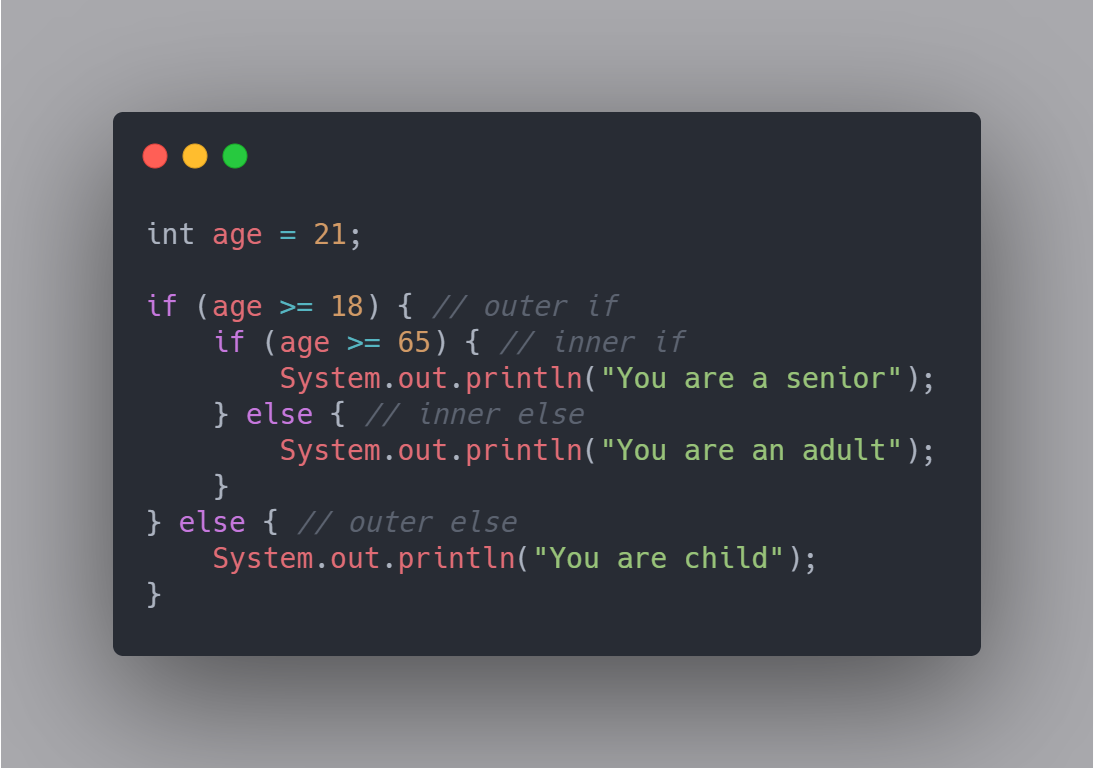
This next concept is called "Nested Ifs". This is when you have if statements inside other if statements. In this example, I have a set of outer if/else statements and a set of inner if/else statements.
So lets step through this program one line at a time. First we are setting our age variable to be equal to 21. Then we are moving on to the if statements. The first if statement is checking if age is 18 or higher. In this case, our user's age is greater
than 18, but if it wasnt, it would automatically go from the outer if to the outer else and execute that print statement. In this case, the program will say the users age is greater than 18 and move onto the inner if statement.
Now it is checking to see if the user's age is 65 or higher. In this case, this would return false and move onto the inner else statement. In the end, this program would print You are an adult
.
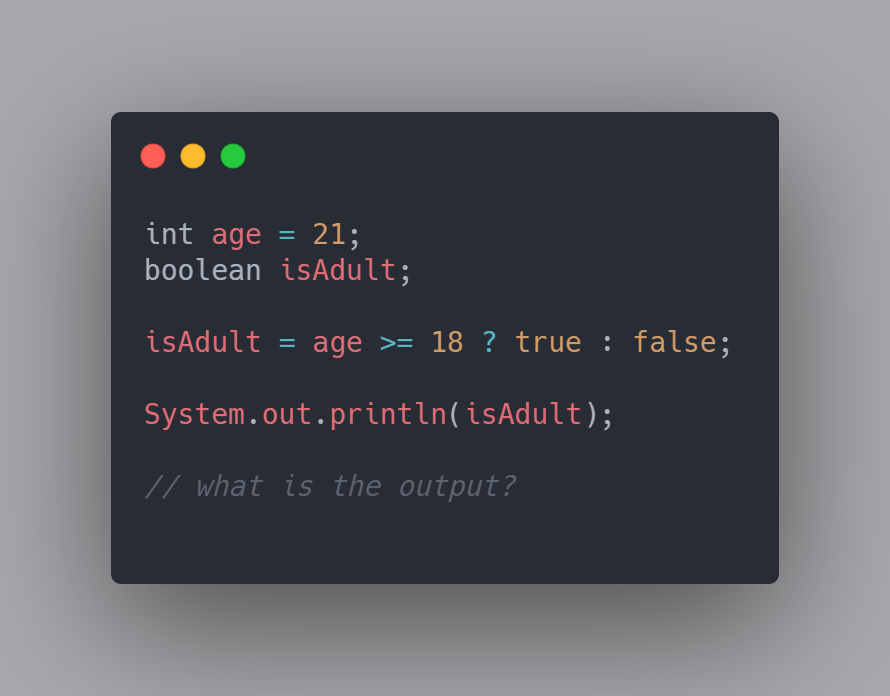
This piece of code is called a "Ternary" statement. This is the final topic we will discuss about how to utilize if statements. So what is a ternary statement? A ternary statement is used as a short hand for an if statement that will produce an if/else. If you have a statement that will always have a true or false outcome, you can use a ternary statement to simplify it.
Lets break down each section of what this ternary statement is doing. Notice at the top, we created a boolean variable called "isAdult" but we never gave it a value. The first portion of the ternary statement is saying where we are going to store the
result, which is inside that variable. So we are saying isAdult =
the result. The next part is what we are actually checking. For consistency, we are doing an age check again using
this code age >= 18
. The question mark ?
is used to end the portion we are checking. Now there is our two sided results true : false;
, the colon seperates it. The left side of the colon
is the result if the check comes back true. The right side is the result if the check comes back false.
So based on this piece of code, what will be printed after the ternary statement is completed?
Congratulations on completing your third lesson in Java! In this lesson we learned how to use if statements. We used if/else as well was if/elseif/else blocks to test multiple conditionals. We also used nested if statements to learn about inner and outer conditionals. Finally we touched on ternary statements for simplified if/else statements. At this point you should have a good idea of how to write small programs that would take in user input as variables, test them in some way with conditionals, and output the result back to the user. I hope you enjoyed this lesson and I'll see you in the next!