Java Basics 101
Lesson 4 - Arrays
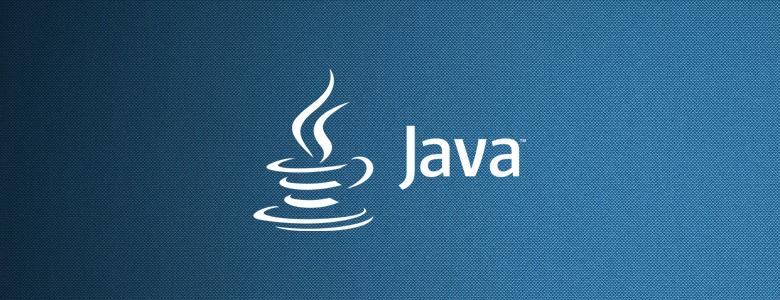
Now that we have covered If statements, we are going to move on to arrays. What is an array? Think of an array like a list of variables. An array means you can have multiple of the same typed variables under a single variable name. You are probably thinking, how can a single variable hold multiple values? Lets get into it and ill show you!
I can index three grades into an array of integers rather than making three variables called “grade1, grade2, grade3”. In the examples, here I will show you how much easier it is to assign variables in an array than individually.
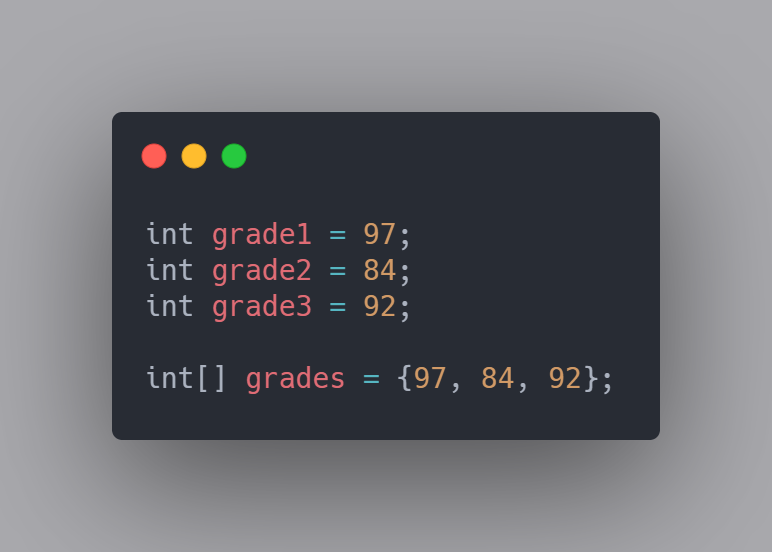
Just by looking at them, you can tell which is more efficient and quicker to write.
Now lets talk about the ways to create and initialize arrays. As you can see in the code, the grades variable has square backets []
attached to the type when creating the variable. This is to signify that this variable is not a single value, but an array of values.
In the example above I had set the variable "grades" to have three values. Just like regular variables, you can create the variable without giving it an intial value. I am going to show you the difference between initializing a variable and an array.
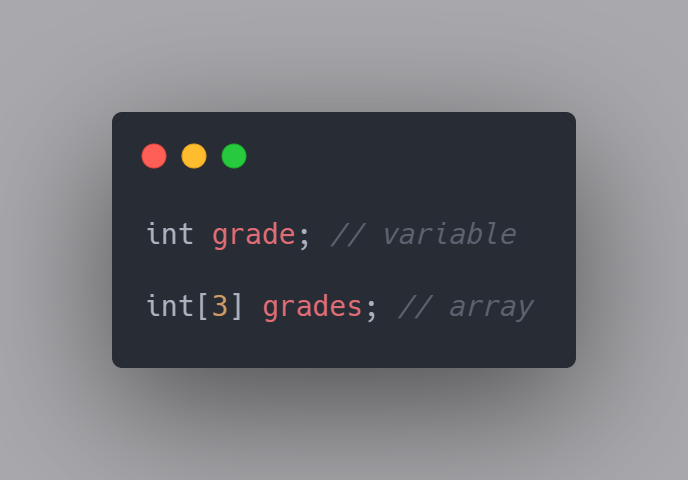
Did you catch the difference? The main difference is still just the []
attached to the variable type to signify an array rather than single variable. What does that number inside the brackets mean though?
Take a guess and then hit the button below.
Lets now take a look at the ways to code an array and give it values.
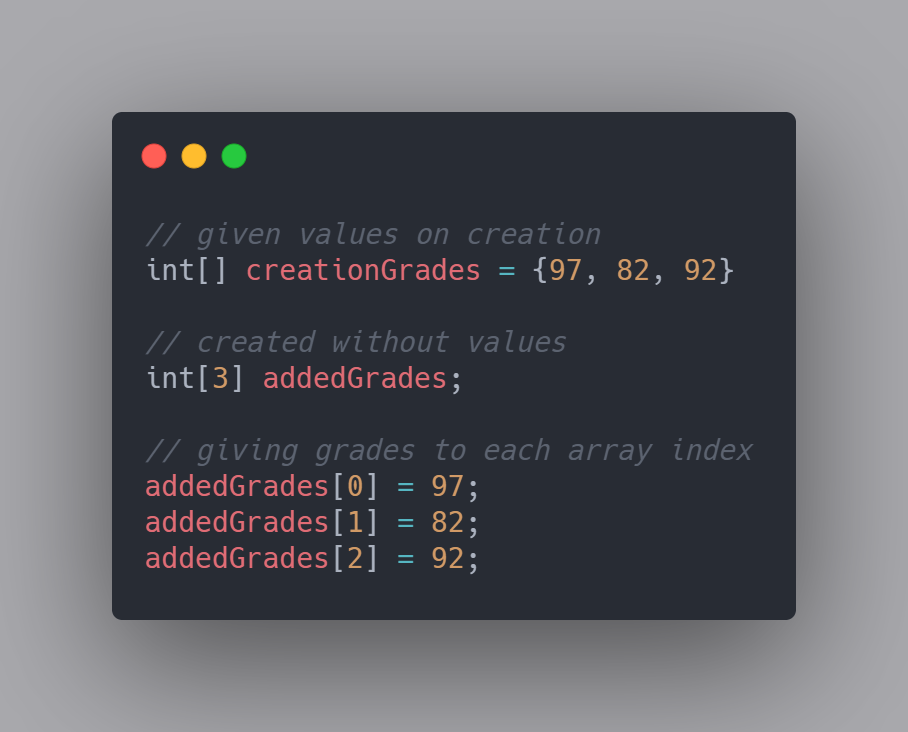
I have given three examples in the code above. In the top example, I knew all the values of the variable from the start, so I was able to input them all right away.
In the middle example, I knew I would have three grades, but I didn't know what they were currently. So I made the array and left the values to be input later on.
In the bottom example, I learned what the values were supposed to be for each grade and input they individually.
Now that we have talked about what arrays are and how to use them, lets discuss some of the pros and cons that arrays have.
Comparison | Variables | Arrays |
---|---|---|
Can change type after creation | No | No |
Number of values can be held at once | Can only hold one value at a time | Can hold as many values as initialized with |
Primitive / Non-Primitive | Primitive (with the exception of string variables) | Non-Primitive |
Now let us discuss these three comparison points!
We will start with the comparison point about changing types. This is one major similarity that regular variables and arrays both share. Once the variable or the array has been created, you cannot change the type of it. If you make an integer variable or an integer array, both will only accept numeric values. Neither an integer variable or an integer array will accept words in their place. There are other types that we will talk about later such as ArrayLists which can take in multiple types.
When comparing how many values a regular variable can have vs an array the answers are very different. With a variable, it will only be able to have one value at a time. The value can be changed multiple times, but it can only ever hold one value at a time. With an array you can hold as many values as you created the array with. It cannot have more or less than it was created with. If you have an array with four seperate numbers and you want to add a fifth, you will have to delete the original array and create a new one with the space to hold five values. If you suddenly want three values instead of four, you will either need to created a new array or you can have a null
value inside one of the array slots.
Finally, lets discuss how most variables are primitive types (with the exception of string variables) and how arrays are non-primitive. So what does primitive and non-primitive mean in Java programming? The difference is whether they have methods or not. So this leads to the question of what is a method? We will discuss it more in depth in a future lesson but the basics of a method is an action that this type can achieve no matter the data entered. Some examples of methods that arrays can utilize in Java include length
, print
, and join
.
I will talk a little about the array methods I mentioned. Length is a method used to return the size of an array. This means if you made the array size of 5, that is what will return when you run the length method. Print is used to iterate over all the values in an array and print them individually. Join is used to join multiple arrays together. This is accomplished by creating a new array of the total size of all the arrays you are joining together. It then will input all the values of the joined arrays into the new array.
In the previous paragraph I mentioned that the exception to the rule of variables being primitive types is the String
variable. Any ideas as to why the string variables are the only variable to be considered non-primitive? Think about what makes them special and then hit the button below for the answer!
Congratulations on completing your fourth lesson in Java! In this lesson we learned how about arrays. We learned how to create arrays with initialized values and how to create them without values and add those later. We talked about the major differences between arrays and variables. We learned about primitive and non-primitive types. We touched on how non-primitive types can have methods and what some examples of these methods are. I hope you enjoyed this lesson and I'll see you in the next!