Java Basics 101
Lesson 1 - Variables and Comments
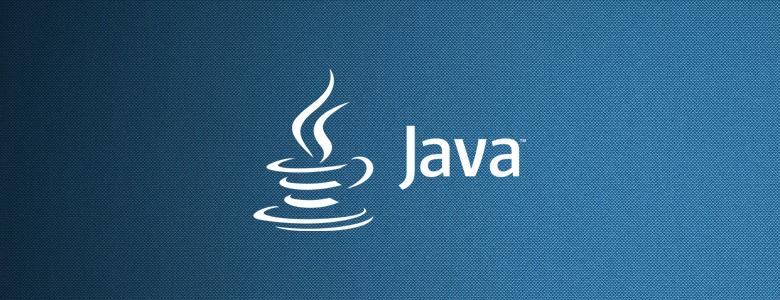
Congratulations on deciding to learn the basics of Java. To start we are going to learn a little bit about what Java is and where it is used. Java is an object-oriented language that was created in the 1990s by James Gosling and colleagues at Sun Microsystems. It was created with the mindset of “write once, run anywhere”. Java is a strong, well maintained language that is not going anywhere anytime soon. As of 2010, Oracle Corporation is the owners and maintainers of Java. It is used in household applications as well as in enterprise level development. Anyway, enough about the history, lets start learning!
So, first off what is a variable? A variable is a named placeholder for a given value. There are many types of variables! There are variable types for true/false values, types for letters and symbols, and others for full sentences. There are also many different variable types for numbers. So, lets go through each one!
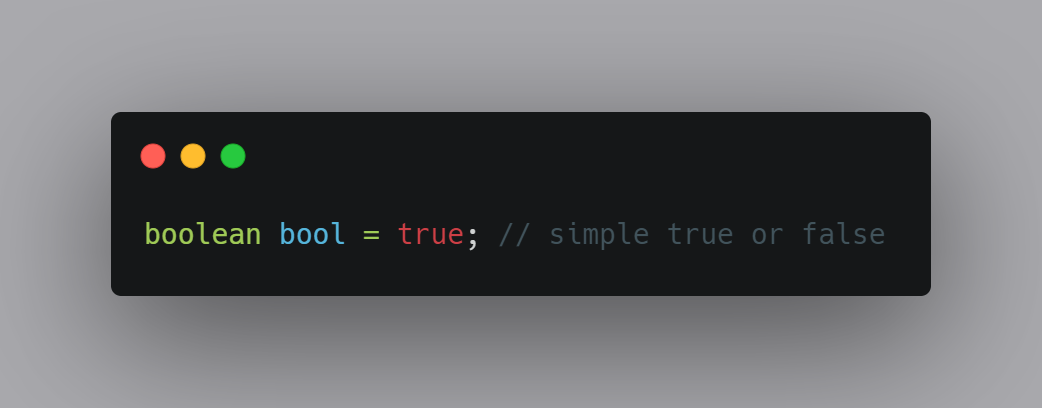
A true or false value is a type “boolean” in Java. This is the most simple type in Java.
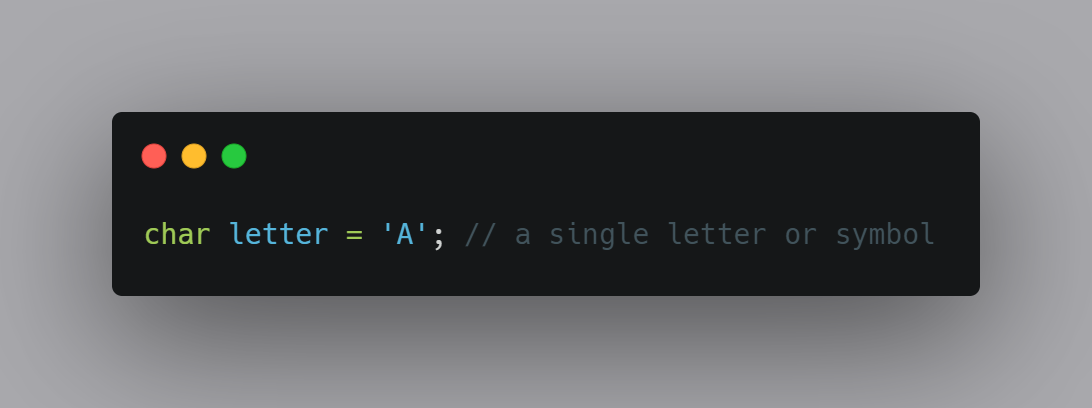
A single letter or symbol uses the type “char” also known as a character. A char can be any individual letter or symbol such as the letter ‘A’ or an ampersand ‘&’.
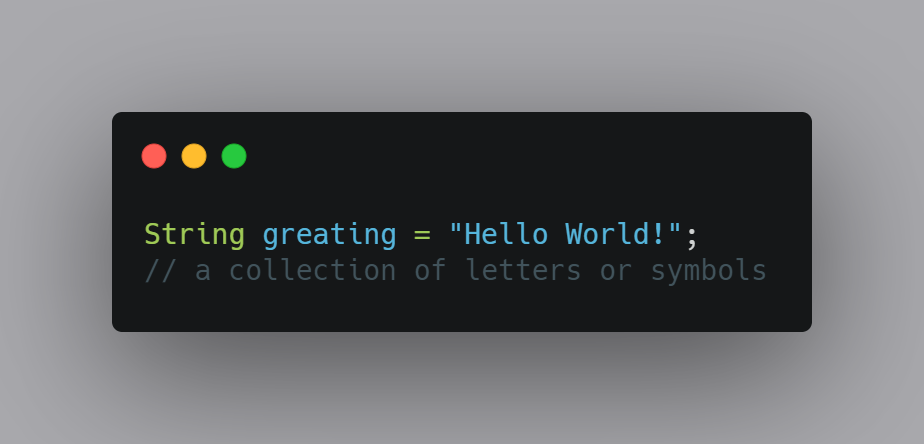
A collection of letters and symbols is called a “string”. A string can hold something simple like “Hello World!” or something a bit more complex such as a Bitcoin public key "12c6DSiU4Rq3P4ZxziKxzrL5LmMBrzjrJX"
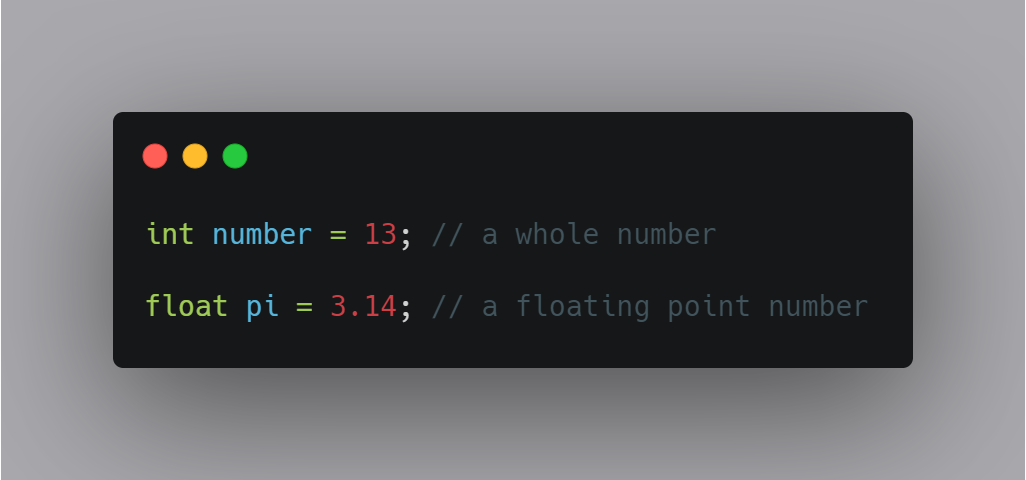
Finally, we will look at numbers! The two main number types we will look at are an “int” or an integer, and a “float” or floating-point number. The main difference between these two types is that an integer will only hold whole numbers such as “5” and “13” whereas a float will hold numbers with decimals such as “3.14” or “123.456”.
There are many other types for numbers that we will not get into, but I wanted to mention “long” and “double”. An integer is a 4-bit whole number whereas a long is an 8-bit whole number. A float is a 4-bit floating-point number whereas a double is an 8-bit floating-point number. So long and double are basically larger versions of integer and float.
The way that a variable is written is by writing the type first, then the name of the variable you wish to use and then saying what it is equal to. Every statement ends with a semi-colon to let the compile know the one statement has been completed. Here is an example of how to write each one of the variables we went over.
Comments are an important part of writing code. It allows the programmer to explain what their thought process for a piece of code. It also allows anyone else reading the code to understand what is being done.
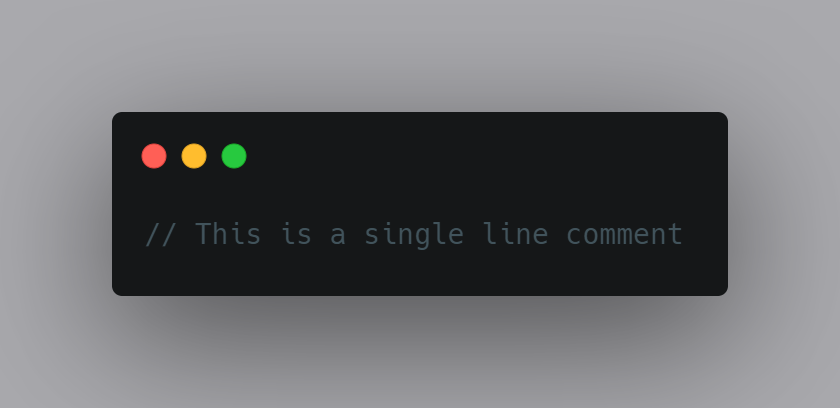
There are two main types of comments in Java. The first is called single line comments. These are done by typing slash-slash (//) and then anything written after will not be read by the computer.
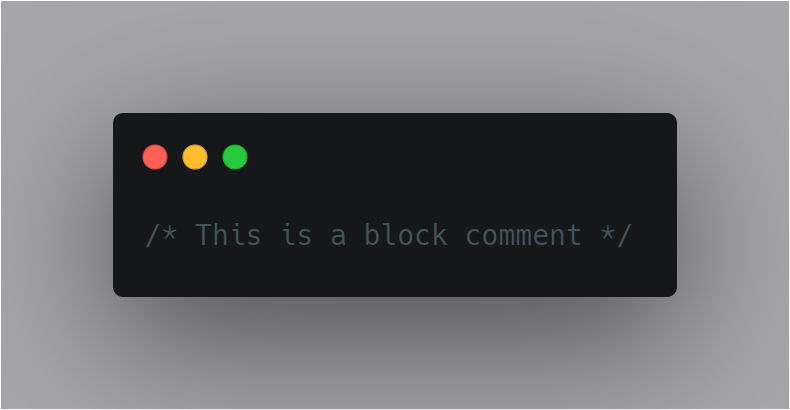
The second type of comment is called a block comment. These are done by typing slash-star (/*) followed by star-slash (*/) and anything inbetween will not be read by the computer.
Congratulations on completing your first lesson in Java! This is your first step in learning and starting your journey with Java and with programming in general. In this lesson we talked about all the different types of variables and what types of values they can hold. We also discussed how to create and initialize the variables with values. We then discussed how to comment your work with different styles of comments and where they would be used. I hope you enjoyed this lesson and I'll see you in the next!